앞서 환경이 구축되었다는 가정하에 Angular 프로젝트를 생성합니다. 터미널에서 ng명령어를 통해 Angular 프로젝트를 생성하고 아래와 같이 서버를 실행합니다.
// ng new 프로젝트명(소문자와 '-'만 이용하여 생성) $ ng new angular-starter ? Would you like to add Angular routing? Yes ? Which stylesheet format would you like to use? CSS CREATE angular-starter/angular.json (3497 bytes) CREATE angular-starter/package.json (1288 bytes) CREATE angular-starter/README.md (1031 bytes) ... 생략 $ cd angular-starter $ ng serve 또는 npm start
브라우저에서는 다음과 같은 화면을 볼수 있습니다.
http://localhost:4200
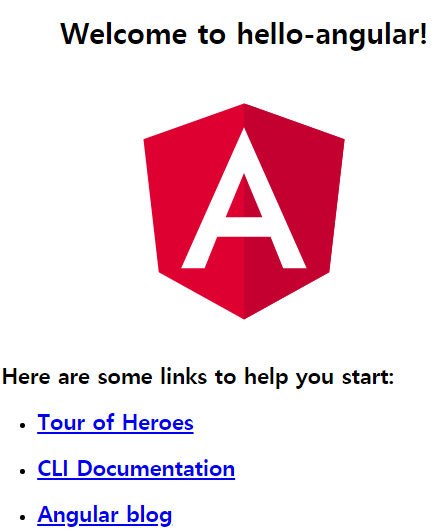
vscode에서 프로젝트 수정
터미널에서 프로젝트 디렉토리로 이동하여 code . 을 입력하면 프로젝트가 선택된 상태로 vscode를 실행할 수 있습니다.
$ cd angular-starter $ code .
다음과 같은 계층 구조의 프로젝트가 생성되는데 대부분의 개발은 src/app 아래에 컴포넌트를 생성하고 개발합니다.
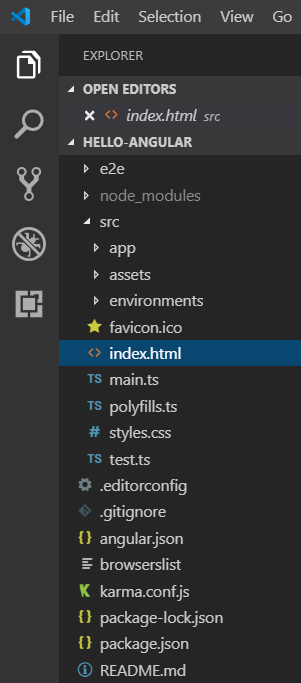
Component
Angular에서의 컴포넌트는 독립적으로 구동 가능한 웹 페이지를 의미합니다. 하나의 웹페이지는 한 개의 컴포넌트나 다수의 컴포넌트로 구성되어 만들어집니다. 다음 명령어로 기본 형태의 컴포넌트를 자동으로 생성할 수 있습니다. 총 4개의 파일이 생성되는데 (*. html, *. css, *. ts, *. spec.ts) *. spec.ts를 제외한 3개의 파일이 컴포넌트를 구성하는 주요 파일입니다.
$ cd src/app $ ng generate component material // 약어로 생성 $ ng g c material CREATE src/app/material/material.component.html (23 bytes) CREATE src/app/material/material.component.spec.ts (642 bytes) CREATE src/app/material/material.component.ts (277 bytes) CREATE src/app/material/material.component.css (0 bytes) UPDATE src/app/app.module.ts (483 bytes)
spec.ts 파일을 생성하고 싶지 않다면 다음 옵션을 추가하여 생성합니다.
$ ng g c material --spec false
컴포넌트는 컴포넌트 내에 중첩하여 사용 가능합니다. 예를 들면 공통 Layout을 구성하기 위해 header, footer, menu 등을 Component로 만들고 레이아웃 Component에서 해당 Component를 include 하여 다양한 레이아웃을 만들어 사용할 수 있습니다.
컴포넌트를 자동으로 생성하면 app.modules.ts 파일 declarations에 자동으로 추가됩니다. 수동으로 컴포넌트를 생성할 경우엔 해당 선언을 수동으로 해줘야 합니다.
declarations: [ AppComponent, MaterialComponent, ..... ]
component ts 파일은 아래와 같은 형식으로 구성됩니다.
@Component({ selector: 'app-material', templateUrl: './material.component.html', styleUrls: ['./material.component.css'] }) export class MaterialComponent implements OnInit { constructor() { } ngOnInit() { } }
selector는 다른 컴포넌트에서 해당 컴포넌트를 include 할 때 사용되는 이름입니다. template url은 컴포넌트의 html 파일 경로입니다. styleUrls은 컴포넌트의 css 파일의 경로입니다.
즉 컴포넌트는 화면에 표시하기 위해 뼈대인 html, 뼈대에 옷을 입히는 css, 그리고 화면에 데이터 및 이벤트를 동적으로 처리하기 위한 ts(typescript) 파일로 구성되어 동작합니다.
Service
서비스는 컴포넌트와 달리 비즈니스 로직만을 처리하기 위한 typescript 파일입니다. 다음 명령어를 통해 자동으로 생성할 수 있습니다. 컴포넌트는 화면까지 처리하기 위해 총 3개의 파일이 필요하지만, service는 비즈니스 로직만을 처리하므로 *. ts 파일 하나만으로 구성됩니다. (spec.ts파일은 테스트용으로 실제 서비스에는 영향을 주지 않습니다.)
$ ng generate service communication // 약어로 생성 $ ng g s communication CREATE src/app/communication.service.spec.ts (368 bytes) CREATE src/app/communication.service.ts (142 bytes)
서비스는 주로 여러 컴포넌트에서 공통으로 사용되는 비즈니스 로직을 처리하기 위해 작성합니다. 예를 들면 외부와의 통신과 같은 부분은 요청과 결과를 제외한 부분은 중복되는 부분이 많은데 이때는 하나의 서비스를 만들어 일관된 통신 방식을 제공하는 것이 효과적입니다.
서비스를 만들면 다른 컴포넌트에서 사용하기 위해 아래와 같이 app.module.ts의 providers에 추가해야 합니다.
providers: [CommunicationService]
서비스를 사용하고자 하는 컴포넌트에서는 constructor안에 선언하고 사용하면 됩니다.
constructor(communicationService: CommunicationService) {}
interpolation, property binding
ts파일에 변수를 선언하면 html파일에서 해당 변수를 사용할 수 있는데 다음과 같이 두가지 방식을 제공합니다. 상황에 따라 적절한 방법을 선택 하여 사용합니다.
// ts 파일 imgSrc= "http://happydaddy.org/happy.jpg"; // html 파일 <img src="{{imgSrc}}" /> // interpolation <img [src]="imgSrc" /> // property binding
Event Binding
기존의 html, javascript 개발 방식은 다음과 같습니다.
<button onclick='onEvent()'>Click</button> <script> function onEvent() { ..... } </script>
Angular에서는 다음과 같이 처리할 수 있습니다. 이벤트 정의는 괄호를 이용해 세팅하며, 이벤트 로직을 ts파일에서 처리합니다.
// html <button (click)="onEvent($event)">Click</button> // ts export class AppComponent { onEvent(event: any) { console.log(event); } }
Event Filtering
이벤트를 처리할때 다수의 이벤트에서 필요한 이벤트만 필터링하여 사용할 수 있습니다. 아래 예제에서는 엔터를 입력하였을때 이벤트 처리하는 방법을 보여주고 있습니다.
// html <input (keyup.enter)="onEnter()"/> // ts export class AppComponent { onEnter() { console.log('Enter Clicked'); } }
Template Variable
#으로 태그안에 이름을 정의하면 다음과 같이 해당 태그 객체에 대한 정보를 이용할수 있습니다.
// html <input #email (keyup.enter)="onSubmit(email.value)"/> // ts export class AppComponent { onSubmit(email) { console.log('Enter value', email); } }
One way, Two way Data binding
단방향 바인딩이란 데이터의 흐름이 한쪽으로만 전달되는 것을 의미합니다. 다음과 같이 ts -> html로 데이터를 바인딩 할 수 있습니다.
// ts name = 'happydaddy'; // html - 화면에 happydaddy가 출력된다. {{name}}
양방향 바인딩이란 데이터의 흐름이 양쪽으로 전달 되는것을 의미합니다. 다음과 같이 ts <-> html 사이의 데이터를 양방향으로 바인딩하여 처리할 수 있습니다.
ngModel을 사용하기 위해 app.module.ts에 FormsModule을 먼저 로드합니다.
import { FormsModule } from '@angular/forms’ imports: [ FormsModule ]
ts에 변수를 선언하고 html input에 해당 변수를 ngModel로 바인딩하면 input값에 따라 ts의 변수값이 변경됩니다. 아래의 예제에서는 {{name}}에는 최초 로드시 HappyDaddy라고 출력이 되는데, input에 값을 입력하면 바인딩된 name의 값이 변경되면서 화면의 {{name}}이 입력된 값으로 치환되어 출력됩니다.
// html {{name}} <input [{ngModel}]='name' /> // ts name = 'HappyDaddy'
Directive
Angular에서는 html 화면에 데이터를 출력하기 위해 조건이나 반복문과 같은 문법을 제공하는데, 그것을 디렉티브라고 합니다.
*ngIf=”조건문”
조건문 안의 내용이 참인 경우에 화면에 표시합니다. 아래의 예제에서는 message 값이 있으면 message를 출력하고 없으면 #other message를 출력합니다.
// ts message = 'Hello Angular'; // html <p *ngIf="message; else other">{{message}}</p> <ng-template #other>There is no message</ng-template>
*ngFor=’let 변수 of 배열명’
배열 사이즈 만큼 루프를 반복하며 ngFor가 선언된 태그를 화면에 반복 출력합니다. 아래 예제에서는 배열 사이즈만큼 루프가 돌면서 화면에 과목명을 출력하게 됩니다.
// ts subjects: string[] = ['국어', '영어', '수학']; // html <ul> <li *ngFor="let subject of subjects"> {{subject}} </li> </ul>
*ngSwitch
주어진 값이 해당하는 경우 처리를 위해 사용합니다. 아래 예제에서는 클릭한 코스에 대한 내용만 화면에 출력하는 예제입니다.
// ts courses: Array<string> = ['Angular', 'Typescript', 'Linux']; coursePage: string; onClick(course) { console.log(course); this.coursePage = course; } // html <div [hidden]="courses.length == 0">Registered courses: <li *ngFor="let course of courses; index as i"> <a (click)="onClick(course)">{{course}}</a> </li> </div> <div [hidden]="courses.length > 0">Not Registered courses</div> <div [ngSwitch]="coursePage"> <div *ngSwitchCase="'Angular'">Angular Page Loding...</div> <div *ngSwitchCase="'Typescript'">Typescript Page Loding...</div> <div *ngSwitchCase="'Linux'">Linux Page Loding...</div> <div *ngSwitchDefault>Default Page Loding...</div> </div>
Pipes
Angular에서는 다음 예제와 같이 출력 데이터에 대해서 추가적인 처리를 한후 화면에 출력할 수 있도록 파이프(‘|’) 명령을 제공합니다. 기본으로 제공되는 몇가지 파이프는 다음과 같으며 자세한 내용은 아래 링크에서 확인할 수 있습니다. https://angular.io/guide/pipes
Built-in pipes
// ts book = { title: 'Lion King', stock: 12345670, star: 4.1256, price: 12000, publishDate: new Date() }; // html {{ book.title | uppercase }}<br> // LION KING {{ book.stock | number }}<br> // 12,345,670 {{ book.star | number: '1.2-2' }}<br> // 4.13 {{ book.price | currency: 'KRW' }}<br> // ₩12,000 {{ book.publishDate | date: 'shortDate' }} // 7/6/19
Custom pipes
ts 파일을 하나 생성하고 다음 내용을 작성합니다. 아래 파이프는 제공된 문자열이 표현하고자 하는 글자수보다 클 경우 줄여서 보여주는 파이프입니다.
// dotdotdot.pipe.ts import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'dotdotdot' }) export class Dotdotdot implements PipeTransform { transform(value: string, limit?: number) { if (!limit) { return null; } return value.substr(0, limit) + '...'; } }
작성한 ts를 app.module.ts의 declarations에 추가합니다.
import { Dotdotdot } from './pipes/dotdotdot.pipe'; declarations: [ Dotdotdot ]
커스텀 pipe의 사용
// ts longText = 'You need to persevere so that when you have done the will of God, you will receive what he has promised.'; // html {{ longText | dotdotdot: '20' }} // You need to persever...
웹페이지 출력
20자까지만 출력되고 나머지 부분은 …으로 대체됩니다.
You need to persever…